/datum
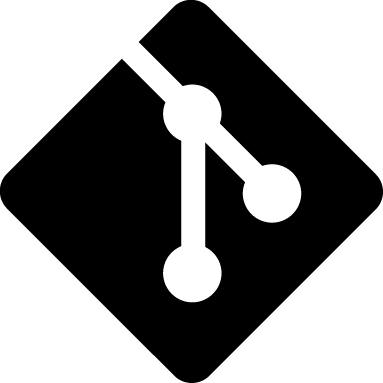
The absolute base class for everything
A datum instantiated has no physical world prescence, use an atom if you want something that actually lives in the world
Be very mindful about adding variables to this class, they are inherited by every single thing in the entire game, and so you can easily cause memory usage to rise a lot with careless use of variables at this level
Vars | |
active_timers | Active timers with this datum as the target |
---|---|
cached_ref | A cached version of our \ref The brunt of \ref costs are in creating entries in the string tree (a tree of immutable strings) This avoids doing that more then once per datum by ensuring ref strings always have a reference to them after they're first pulled |
comp_lookup | Any datum registered to receive signals from this datum is in this list |
datum_components | Components attached to this datum |
datum_flags | Datum level flags |
gc_destroyed | Tick count time when this object was destroyed. |
signal_enabled | Is this datum capable of sending signals? |
signal_procs | Lazy associated list in the structure of signals:proctype that are run when the datum receives that signal |
status_traits | Status traits attached. |
tgui_shared_states | global |
weak_reference | A weak reference to another datum |
Procs | |
Destroy | Default implementation of clean-up code. |
GetComponent | Return any component assigned to this datum of the given type |
GetComponents | Get all components of a given type that are attached to this datum |
GetExactComponent | Return any component assigned to this datum of the exact given type |
LoadComponent | Get existing component of type, or create it and return a reference to it |
RegisterSignal | Register to listen for a signal from the passed in target |
TakeComponent | Transfer this component to another parent |
TransferComponents | Transfer all components to target |
UnregisterSignal | Stop listening to a given signal from target |
_AddComponent | Creates an instance of new_type in the datum and attaches to it as parent |
_AddElement | Finds the singleton for the element type given and attaches it to src |
_RemoveElement | Finds the singleton for the element type given and detaches it from src You only need additional arguments beyond the type if you're using ELEMENT_BESPOKE |
_SendSignal | Internal proc to handle most all of the signaling procedure |
p_they | NAMEOF that actually works in static definitions because src::type requires src to be defined |
process | This proc is called on a datum on every "cycle" if it is being processed by a subsystem. The time between each cycle is determined by the subsystem's "wait" setting. You can start and stop processing a datum using the START_PROCESSING and STOP_PROCESSING defines. |
tgui_interact | public |
ui_act | public |
ui_assets | public |
ui_close | public |
ui_data | public |
ui_host | private |
ui_state | private |
ui_static_data | public |
ui_status | public |
update_static_data | public |
update_static_data_for_all_viewers | public |
vv_edit_var | Called whenever a var is edited to edit the var, returning FALSE will reject the edit. |
Var Details
active_timers
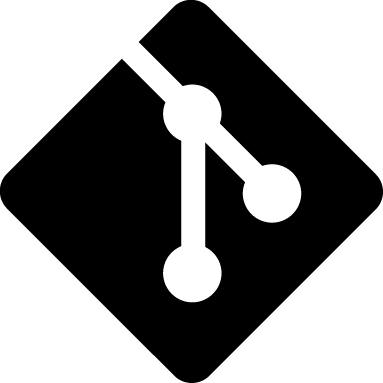
Active timers with this datum as the target
cached_ref
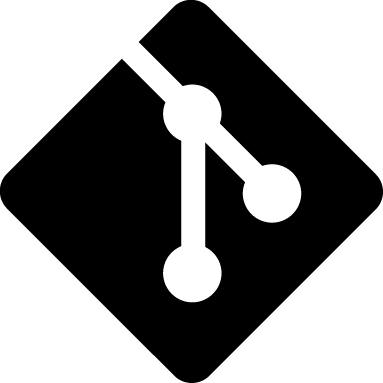
A cached version of our \ref The brunt of \ref costs are in creating entries in the string tree (a tree of immutable strings) This avoids doing that more then once per datum by ensuring ref strings always have a reference to them after they're first pulled
comp_lookup
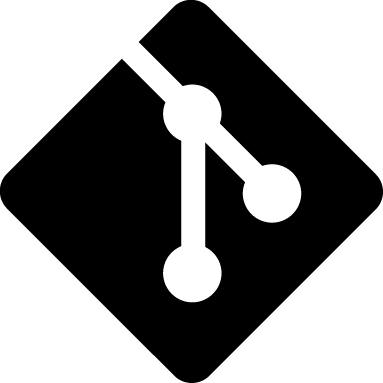
Any datum registered to receive signals from this datum is in this list
Lazy associated list in the structure of signal:registree/list of registrees
datum_components
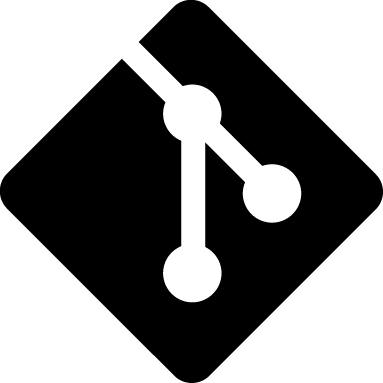
Components attached to this datum
Lazy associated list in the structure of type:component/list of components
datum_flags
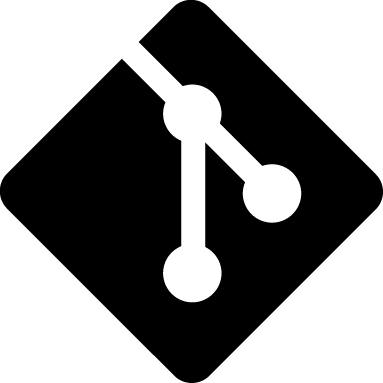
Datum level flags
gc_destroyed
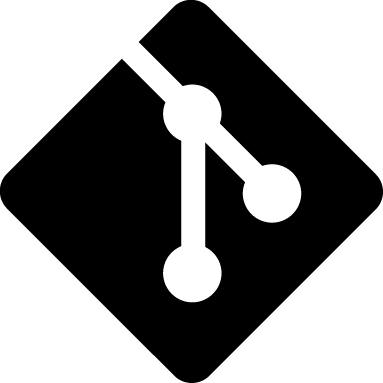
Tick count time when this object was destroyed.
If this is non zero then the object has been garbage collected and is awaiting either a hard del by the GC subsystme, or to be autocollected (if it has no references)
signal_enabled
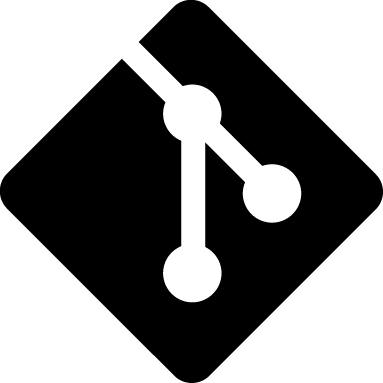
Is this datum capable of sending signals?
Set to true when a signal has been registered
signal_procs
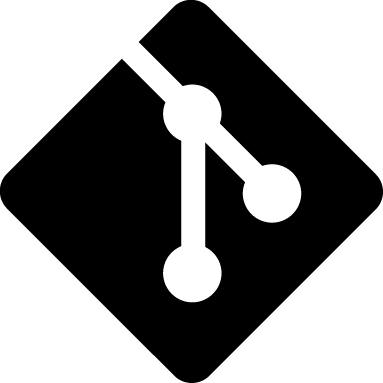
Lazy associated list in the structure of signals:proctype
that are run when the datum receives that signal
status_traits
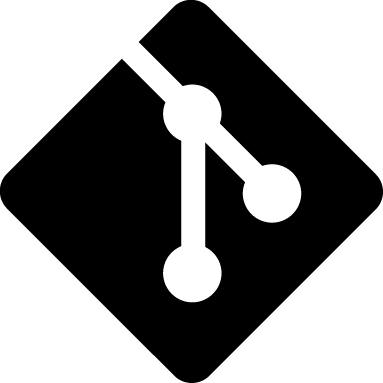
Status traits attached.
tgui_shared_states
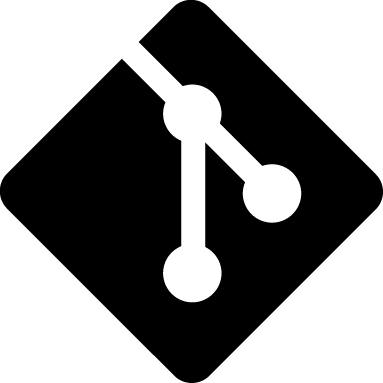
global
Associative list of JSON-encoded shared states that were set by tgui clients.
weak_reference
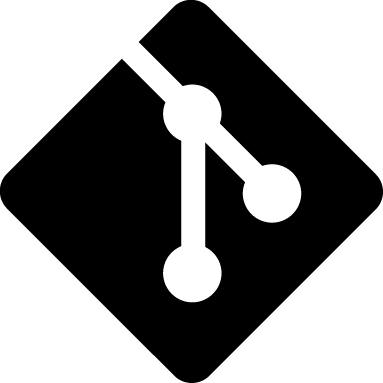
A weak reference to another datum
Proc Details
Destroy
Default implementation of clean-up code.
This should be overridden to remove all references pointing to the object being destroyed, if you do override it, make sure to call the parent and return it's return value by default
Return an appropriate QDEL_HINT to modify handling of your deletion; in most cases this is QDEL_HINT_QUEUE.
The base case is responsible for doing the following
- Erasing timers pointing to this datum
- Erasing compenents on this datum
- Notifying datums listening to signals from this datum that we are going away
Returns QDEL_HINT_QUEUE
GetComponent
Return any component assigned to this datum of the given type
This will throw an error if it's possible to have more than one component of that type on the parent
Arguments:
- datum/component/c_type The typepath of the component you want to get a reference to
GetComponents
Get all components of a given type that are attached to this datum
Arguments:
- c_type The component type path
GetExactComponent
Return any component assigned to this datum of the exact given type
This will throw an error if it's possible to have more than one component of that type on the parent
Arguments:
- datum/component/c_type The typepath of the component you want to get a reference to
LoadComponent
Get existing component of type, or create it and return a reference to it
Use this if the item needs to exist at the time of this call, but may not have been created before now
Arguments:
- component_type The typepath of the component to create or return
- ... additional arguments to be passed when creating the component if it does not exist
RegisterSignal
Register to listen for a signal from the passed in target
This sets up a listening relationship such that when the target object emits a signal the source datum this proc is called upon, will receive a callback to the given proctype Return values from procs registered must be a bitfield
Arguments:
- datum/target The target to listen for signals from
- sig_type_or_types Either a string signal name, or a list of signal names (strings)
- proctype The proc to call back when the signal is emitted
- override If a previous registration exists you must explicitly set this
TakeComponent
Transfer this component to another parent
Component is taken from source datum
Arguments:
- datum/component/target Target datum to transfer to
TransferComponents
Transfer all components to target
All components from source datum are taken
Arguments:
- /datum/target the target to move the components to
UnregisterSignal
Stop listening to a given signal from target
Breaks the relationship between target and source datum, removing the callback when the signal fires
Doesn't care if a registration exists or not
Arguments:
- datum/target Datum to stop listening to signals from
- sig_typeor_types Signal string key or list of signal keys to stop listening to specifically
_AddComponent
Creates an instance of new_type
in the datum and attaches to it as parent
Sends the COMSIG_COMPONENT_ADDED signal to the datum
Returns the component that was created. Or the old component in a dupe situation where COMPONENT_DUPE_UNIQUE was set
If this tries to add a component to an incompatible type, the component will be deleted and the result will be null
. This is very unperformant, try not to do it
Properly handles duplicate situations based on the dupe_mode
var
_AddElement
Finds the singleton for the element type given and attaches it to src
_RemoveElement
Finds the singleton for the element type given and detaches it from src You only need additional arguments beyond the type if you're using ELEMENT_BESPOKE
_SendSignal
Internal proc to handle most all of the signaling procedure
Will runtime if used on datums with an empty component list
Use the SEND_SIGNAL define instead
p_they
NAMEOF that actually works in static definitions because src::type requires src to be defined
process
This proc is called on a datum on every "cycle" if it is being processed by a subsystem. The time between each cycle is determined by the subsystem's "wait" setting. You can start and stop processing a datum using the START_PROCESSING and STOP_PROCESSING defines.
Since the wait setting of a subsystem can be changed at any time, it is important that any rate-of-change that you implement in this proc is multiplied by the delta_time that is sent as a parameter, Additionally, any "prob" you use in this proc should instead use the DT_PROB define to make sure that the final probability per second stays the same even if the subsystem's wait is altered. Examples where this must be considered:
- Implementing a cooldown timer, use
mytimer -= delta_time
, notmytimer -= 1
. This way,mytimer
will always have the unit of seconds - Damaging a mob, do
L.adjustFireLoss(20 * delta_time)
, notL.adjustFireLoss(20)
. This way, the damage per second stays constant even if the wait of the subsystem is changed - Probability of something happening, do
if(DT_PROB(25, delta_time))
, notif(prob(25))
. This way, if the subsystem wait is e.g. lowered, there won't be a higher chance of this event happening per second
If you override this do not call parent, as it will return PROCESS_KILL. This is done to prevent objects that dont override process() from staying in the processing list
tgui_interact
public
Used to open and update UIs. If this proc is not implemented properly, the UI will not update correctly.
required user mob The mob who opened/is using the UI. optional ui datum/tgui The UI to be updated, if it exists.
ui_act
public
Called on a UI when the UI receieves a href. Think of this as Topic().
required action string The action/button that has been invoked by the user. required params list A list of parameters attached to the button.
return bool If the user's input has been handled and the UI should update.
ui_assets
public
Called on an object when a tgui object is being created, allowing you to push various assets to tgui, for examples spritesheets.
return list List of asset datums or file paths.
ui_close
public
Called on a UI's object when the UI is closed, not to be confused with client/verb/uiclose(), which closes the ui window
ui_data
public
Data to be sent to the UI. This must be implemented for a UI to work.
required user mob The mob interacting with the UI.
return list Data to be sent to the UI.
ui_host
private
The UI's host object (usually src_object). This allows modules/datums to have the UI attached to them, and be a part of another object.
ui_state
private
The UI's state controller to be used for created uis This is a proc over a var for memory reasons
ui_static_data
public
Static Data to be sent to the UI.
Static data differs from normal data in that it's large data that should be sent infrequently. This is implemented optionally for heavy uis that would be sending a lot of redundant data frequently. Gets squished into one object on the frontend side, but the static part is cached.
required user mob The mob interacting with the UI.
return list Statuic Data to be sent to the UI.
ui_status
public
Checks the UI state for a mob.
required user mob The mob who opened/is using the UI. required state datum/ui_state The state to check.
return UI_state The state of the UI.
update_static_data
public
Forces an update on static data. Should be done manually whenever something happens to change static data.
required user the mob currently interacting with the ui optional ui ui to be updated
update_static_data_for_all_viewers
public
Will force an update on static data for all viewers. Should be done manually whenever something happens to change static data.
vv_edit_var
Called whenever a var is edited to edit the var, returning FALSE will reject the edit.